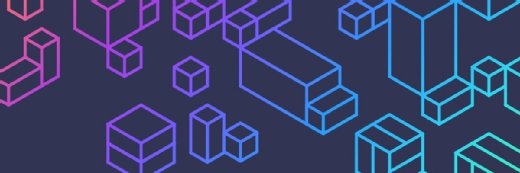
Getty Images
Using PowerShell for monitoring SharePoint Online
The scale and complexity of some SharePoint Online environments can make maintenance difficult. Learn to use automation scripts to keep tabs on the collaboration platform.
SharePoint Online is a powerful cloud-based collaboration and data management platform in Microsoft 365. It's imperative to check that SharePoint Online works optimally by monitoring its activities and site health.
Many organizations depend on SharePoint Online to store, share and manage content, workflows and business processes. As the administrator, you need an efficient and reliable method to monitor SharePoint Online activities to maintain the platform's integrity and performance. This article explains how to use PowerShell's automation capabilities to build scripts to perform these checks and gather detailed insights into site usage to also ensure the organization adheres to security and compliance requirements.
How to connect to SharePoint Online with PowerShell
The first step to monitoring SharePoint Online with PowerShell is to connect the cloud service to the automation tool.
The Microsoft.Online.SharePoint.PowerShell module, which Microsoft also calls the Microsoft SharePoint Online Services module, is a set of PowerShell cmdlets provided by Microsoft for SharePoint Online management. These cmdlets perform various administrative tasks from the command line.
First, install the module on your system with the following command:
Install-Module -Name Microsoft.Online.SharePoint.PowerShell -Force
This is for Windows PowerShell.
You can load the module within PowerShell 7.x using the Windows PowerShell Compatibility feature:
Import-Module -Name Microsoft.Online.SharePoint.PowerShell -UseWindowsPowerShell
This command forces the module to load using Windows PowerShell, even if you use PowerShell 7. Not all cmdlets may work as expected due to the underlying differences between the newer cross-platform PowerShell and Windows PowerShell.
Next, connect to your SharePoint Online tenant. Replace the URL in the following code with your SharePoint admin center URL. This example prompts for your Microsoft 365 credentials and connects you to the SharePoint Online service:
Connect-SPOService -Url https://tenant-admin.sharepoint.com
The Patterns and Practices (PnP) PowerShell module offers a more versatile and feature-rich approach for managing SharePoint Online. It provides a library of PowerShell commands designed to perform complex tasks on the platform more efficiently. Install the PnP PowerShell module with the following commands:
Install-Module PnP.PowerShell -Force
Register-PnPManagementShellAccess
Next, connect to your SharePoint Online site. In the following example, replace $SiteURL with the URL of your SharePoint Online site. This command also prompts for credentials and connects you to the specified SharePoint Online site:
$SiteURL = "https://tenant-admin.sharepoint.com"
Connect-PnPOnline $SiteURL -Interactive
Both the Microsoft SharePoint Online Services and PnP PowerShell modules are good options for administrators to connect to and manage SharePoint Online via PowerShell. There are different capabilities in each module. Regular updates and a strong community make PnP PowerShell the preferred PowerShell management module for many administrators, but the official Microsoft module also has its strengths, especially in basic site management tasks. Knowing how to use each module provides the best coverage.
How to use PowerShell to check SharePoint site usage
To start with basic site usage details, such as storage used and last content modified date, you can use the Get-SPOSite cmdlet:
$siteUrl = "https://m365x86127502.sharepoint.com/sites/ContosoNews"
$site = Get-SPOSite -Identity $siteUrl -Detailed
$siteInfo = @"
URL: $($site.Url)
Storage Used: $($site.StorageUsageCurrent) MB
Last Content Modified: $($site.LastContentModifiedDate)
"@
Write-Host $siteInfo
SharePoint Online has storage limits, making it important to understand the storage use of your site collections to avoid errors or paying higher fees for added space. This command lists all site collections with their current storage usage in megabytes:
Get-SPOSite -Limit All | `
Select-Object Url, StorageUsageCurrent | `
Format-Table -AutoSize
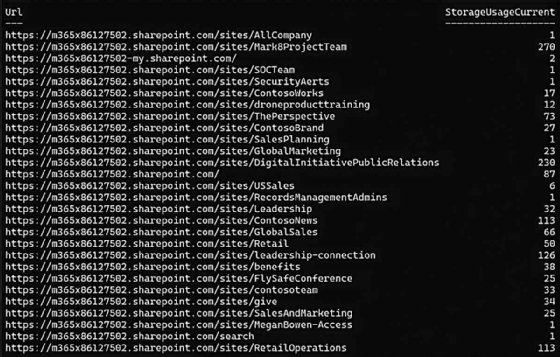
Audit logs in SharePoint Online reveal insights into user activities, document access and system operations. While the Exchange Online PowerShell module -- also called the Exchange Online PowerShell V3 module or the EXO V3 module -- is primarily for managing Exchange Online, it can also retrieve SharePoint Online site information through audit logs, indirectly indicating site health. The Search-UnifiedAuditLog cmdlet in the EXO V3 module provides access to the unified audit log in Microsoft 365.
First, install the Exchange Online PowerShell module if you haven't already:
Install-Module -Name ExchangeOnlineManagement -Force
Import-Module -Name ExchangeOnlineManagement
Next, use your credentials to connect to the Exchange Online service. Ensure that the account used has the necessary permissions to access audit logs:
Connect-ExchangeOnline `
-UserPrincipalName [email protected]
After establishing the connection, use the Search-UnifiedAuditLog cmdlet to retrieve audit log entries for SharePoint activities from the last 30 days:
$searchParams = @{
StartDate = (Get-Date).AddDays(-30)
EndDate = Get-Date
RecordType = "SharePoint"
}
Search-UnifiedAuditLog @searchParams | `
Select-Object Operations, AuditData | Format-Table
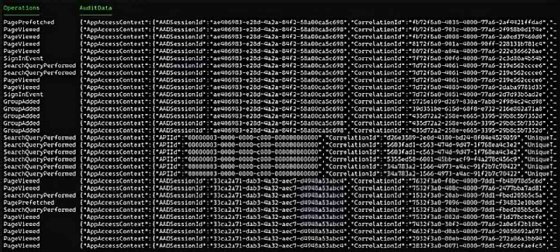
How to monitor user engagement in SharePoint Online
Understanding user engagement on SharePoint Online sites, such as page views, file downloads and user interactions, helps the organization optimize UX and manage content more effectively.
Microsoft SharePoint Online Services or any other standard PowerShell module for SharePoint Online does not have cmdlets to monitor file downloads. Instead, you use the SharePoint Online audit logs through the security and compliance center. You can also use the Search-UnifiedAuditLog cmdlet in the Exchange Online PowerShell module to monitor these activities.
The following commands retrieve file downloads within SharePoint Online during the past 30 days:
$searchParams = @{
StartDate = (Get-Date).AddDays(-90)
EndDate = Get-Date
RecordType = "SharePointFileOperation"
}
$auditLogs = Search-UnifiedAuditLog @searchParams
$fileDownloads = $auditLogs | `
Where-Object { $_.Operations -contains "FileDownloaded" }
$fileDownloads | `
Select-Object CreationDate, UserIds, Operations, AuditData | `
Format-Table
By analyzing user interactions, file downloads and page views, the administrator can share findings with stakeholders who want to know the level of user engagement. The following script uses the PnP PowerShell module to output file access activities:
$auditLogs = Get-PnPUnifiedAuditLog `
-ContentType "SharePoint"
$auditLogs | `
Where-Object { $_.Operation -contains "FileAccessed" } | `
Select-Object Operation, SiteUrl, SourceFileName, Workload | `
Format-Table
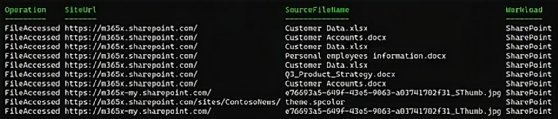
How to audit user activity on SharePoint Online sites
User activity reports are critical for understanding which users interact with the SharePoint environment and how. The following example lists all users of a particular site, excluding site admins:
$siteUrl = "https://m365x86127502.sharepoint.com/sites/ContosoNews"
$siteUsageUsers = Get-SPOUser -Site $siteUrl
$siteUsageUsers | `
Where-Object {$_.IsSiteAdmin -eq $false} | `
Select-Object DisplayName, UserType | `
Format-Table -AutoSize
Either of the PowerShell modules can return more advanced usage metrics, such as page views and unique viewers. The following PowerShell code filters the audit logs for page view events and displays the results in a table:
$auditLogs = Get-PnPUnifiedAuditLog `
-ContentType "SharePoint"
$pageViewLogs = $auditLogs | `
Where-Object { $_.Operation -eq "PageViewed" } | `
Select-Object UserKey, Operation, Workload | `
Format-Table
$pageViewLogs
Monitoring file and folder activities gives admins a way to ensure the organization meets its compliance requirements and also helps detect suspicious activity on the SharePoint Online platform. The following PowerShell code checks SharePoint for file changes and uploads in the last 30 days and outputs the results:
$searchParams = @{
StartDate = (Get-Date).AddDays(-30)
EndDate = Get-Date
RecordType = "SharePointFileOperation"
Operations = "FileModified", "FileUploaded"
}
Search-UnifiedAuditLog @searchParams | `
Format-Table

How to check for user permissions changes in SharePoint Online
Using PowerShell automation to track changes in user permissions in SharePoint Online helps to avoid security and compliance issues. While the Microsoft 365 admin center has some level of tracking, PowerShell can uncover more comprehensive information.
The following script checks the unified audit log for group and permissions changes in SharePoint Online:
$searchParams = @{
StartDate = (Get-Date).AddDays(-30)
EndDate = Get-Date
RecordType = "SharePoint"
Operations = "AddedToGroup",
"RemovedFromGroup", "UpdatedGroup", "SharePointGroupOperation"
}
$auditLogs = Search-UnifiedAuditLog @searchParams
$auditLogs | Select-Object UserIds, Operations, AuditData | `
Format-Table
How to check SharePoint Online site health
Using PowerShell to monitor SharePoint Online offers a quick way to examine changes in key areas of the platform to make sure users follow the policies and regulations of the organization.
The following example uses the Microsoft SharePoint Online Services module to retrieve the status of a specified site collection, indicating its health.
$site = Get-SPOSite -Identity $siteUrl -Detailed
Write-Host "Status: $($site.Status)"
The following script uses the SharePoint Online audit log to show how many operations a user performs. These operations include activities related to file and folder modifications, permissions changes, and list and list item access:
$searchParams = @{
StartDate = (Get-Date).AddDays(-30)
EndDate = Get-Date
RecordType = "SharePoint"
}
$auditLogs = Search-UnifiedAuditLog @searchParams
$userOperationsCount = $auditLogs | `
Group-Object -Property UserIds -NoElement |
Select-Object @{Name="User";Expression={($_.Name -join ', ')}}, @{Name="OperationsCount";Expression={$_.Count}}
$userOperationsCount | Format-Table -AutoSize
Try PowerShell to optimize SharePoint Online oversight
Automation via PowerShell is one way to customize your own monitoring tool to manage and maintain SharePoint Online.
With the Microsoft SharePoint Online Services module, EXO V3 module and PnP PowerShell module, administrators can tailor their management strategies to their organization's needs through detailed auditing and proactive site health management.
PowerShell gives administrators who manage SharePoint Online a way to enhance oversight and streamline operations for a healthier and more secure environment. As SharePoint Online and PowerShell evolve, administrators must stay updated with the latest developments to take full advantage of these powerful tools.
Liam Cleary is founder and owner of SharePlicity, a technology consulting company that helps organizations with internal and external collaboration, document and records management, business process automation, automation tool deployment, and security controls and protection. Cleary's areas of expertise include security on the Microsoft 365 and Azure platforms, PowerShell automation and IT administration. Cleary is a Microsoft MVP and a Microsoft Certified Trainer.