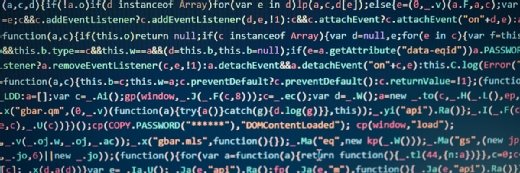
Getty Images
How to write pseudocode: A guided tutorial
Writing pseudocode might feel like an extra step, but its value becomes clear in complex projects. Focus on the logic without getting tangled in language-specific syntax.
Every solid software implementation starts with systematic planning.
Before diving into code, programmers need a way to substantiate logic without getting caught up in a specific programming language's syntax. This detailed guide, featuring a plan for a basic API rate limiter and other real-world examples, can assist you in crafting clear, functional and effective pseudocode to tackle any programming challenge.
Core pseudocode constructs
Programming logic flows through sequences, decisions and loops. The following pseudocode constructs, each supplemented by a general workflow example, form the basis of algorithmic problem-solving and enable developers to model real-world processes -- from edge case troubleshooting in algorithm behavior to creating phased implementation roadmaps that balance technical debt with feature priorities.
Sequential operations
A program starts with steps that execute one after another. While this might seem obvious, mastering sequential flow, or SEQUENCE, in pseudocode is essential for addressing more complex logic. Consider the following user registration workflow and notice how sequential operations dictate the mandatory steps:
INPUT user_data
VALIDATE user_data
PROCESS user_data
OUTPUT results
Decision making
Program flow rarely follows a linear path. It requires branching logic through IF-THEN-ELSE constructs to create the dynamic behavior, like the following age-gated content access:
IF age < 18 THEN
DISPLAY "Access denied"
ELSE
GRANT access
ENDIF
Different conditional scenarios call for different approaches. Use CASE statements to clarify logic and intent when multiple conditions are involved. For example, the following workflow can process different payment methods depending on the input:
CASE payment_method OF
"credit": PROCESS credit_payment
"debit": PROCESS debit_payment
"crypto": PROCESS crypto_payment
ENDCASE
CASE statements are optimized for evaluating multiple values of a single variable, whereas IF statements are more suitable for Boolean conditions and complex logical expressions involving multiple variables. Additionally, compiler optimizations can execute CASE statements faster through jump tables.
Loops and iterations
Loops automate repetitive tasks by executing code blocks multiple times based on specified conditions. They're essential for processing data collections, running calculations iteratively or maintaining continuous operations. FOR loops are the best choice when the exact number of iterations is known, as demonstrated in the following shopping cart calculation:
FOR each item IN shopping_cart
CALCULATE item_total
ADD TO cart_total
ENDFOR
But what about dynamic conditions? WHILE loops can effectively handle these scenarios, as shown in the following network connection example:
WHILE connection_active
PROCESS incoming_data
CHECK connection_status
ENDWHILE
REPEAT-UNTIL loops offer similar functionality to WHILE but guarantee at least one execution since they check the condition after running the code block, as illustrated in the following input validation example:
REPEAT
GET user_input
VALIDATE input
UNTIL input_valid
Function handling
Functions break down complex operations into smaller, digestible chunks. They accept parameters, process data and return results while maintaining scope isolation. Consider the following pseudocode for validating user credentials:
FUNCTION validate_user(username, password)
CHECK username_exists
VERIFY password_hash
RETURN validation_status
ENDFUNCTION
Exception management
Runtime errors are inevitable when processing user input, accessing external resources or performing complex calculations. TRY-CATCH blocks can help plan for potential errors, as shown in the following example that flags an anomaly when processing sensitive data and notifies the admin of it:
TRY
PROCESS sensitive_data
CATCH data_error
LOG error_details
NOTIFY admin
ENDTRY
Key guidelines for writing pseudocode
Effective pseudocode balances expressiveness with readability, clearly communicating intent while remaining implementation-agnostic. Here are some tips and general best practices for optimizing the pseudocode process:
Syntax guidelines
- Write keywords in UPPERCASE. Keywords serve as signposts of the code's logic. WHILE, IF, FUNCTION -- these stand out visually and help clarify flow.
- Place each statement on its own line.
Naming conventions
- Skip cryptic variable names and use_descriptive_names that tell the data's story. Whether it's customer_record or temp_buffer, make what the code entails obvious.
- Stick to action verbs for functions, such as calculate_total(), validate_input() or process_transaction().
Structure and flow
- Keep statements concise but complete. Pseudocode should read like a well-written technical document. Instead of something like "x = x + 1", write "INCREMENT counter BY 1" to maintain language independence.
- Use proper indentation to indicate the logical hierarchy of the algorithm and nest constructs thoughtfully. Each level of indentation signals a new logical scope, as illustrated here:
IF user_authenticated THEN
FOR each_permission IN user_permissions
IF permission_active THEN
GRANT access_level
ENDIF
ENDFOR
ENDIF
- Explicitly close constructs with ENDIF, ENDWHILE or ENDFOR. These markers prevent logical leaks and make the pseudocode self-documenting.
How to write pseudocode: Practical applications
Pseudocode might be overkill for simple CRUD operations or straightforward implementations, but it is a valuable communication tool for algorithmic challenges, system design or even team discussions. The following examples demonstrate building an API rate limiter and writing a bubble sort algorithm using pseudocode.
Build an API rate limiter with pseudocode
Rate limiting might seem simple on the surface -- just count requests and block incoming requests when they exceed a certain threshold. But dig deeper, and it's possible to find a maze of edge cases that can bring down an API if not properly handled.
Let's create a rate limiter using pseudocode that caps users at 100 requests per hour. This implementation will also consider distributed systems, race conditions and temporary traffic spikes.
First, the core rate-checking logic:
FUNCTION check_rate_limit(user_id)
DEFINE requests_limit = 100
DEFINE time_window = 3600 // seconds in an hour
GET current_timestamp
REMOVE expired_requests(user_id, current_timestamp - time_window)
GET request_count FOR user_id IN time_window
IF request_count >= requests_limit THEN
RETURN rate_limit_exceeded
ENDIF
ADD new_request(user_id, current_timestamp)
RETURN request_accepted
ENDFUNCTION
In a distributed system, multiple servers might try updating the same user's request count simultaneously. Atomic operations can prevent race conditions.
FUNCTION add_new_request(user_id, timestamp)
TRY
LOCK user_requests_table
INCREMENT request_counter
ADD request_record(user_id, timestamp)
RELEASE lock
CATCH concurrency_error
HANDLE distributed_system_error
RETURN system_busy
ENDTRY
ENDFUNCTION
But how to manage traffic spikes? Simple counters won't cut it when an API faces sudden request surges. For finer control over API traffic, use a layered approach that combines fixed rate limits with burst handling. The burst handler acts as a pressure valve, preventing legitimate traffic spikes from being incorrectly throttled while protecting the system from abuse.
FUNCTION handle_burst_traffic(user_id, timestamp)
GET current_window_stats
IF request_spike_detected THEN
CALCULATE burst_allowance
IF within_burst_limits THEN
INCREMENT burst_counter
RETURN request_accepted
ENDIF
ENDIF
RETURN regular_rate_check(user_id)
ENDFUNCTION
Bubble sort with pseudocode
A bubble sort algorithm is a way to order numbers in a list by comparing them. Here's how a developer might write a sorting algorithm if they're in a rush:
function sort(arr)
n = length(arr)
for i = 0 to n
for j = 0 to n
if arr[j] > arr[j+1]
t = arr[j]
arr[j] = arr[j+1]
arr[j+1] = t
return arr
Notice any issues? There are missing bounds checks, unclear variable names, no error handling and incorrect loop termination conditions. This implementation could lead to array index errors.
Here's a more structured implementation of the same bubble sort:
FUNCTION bubble_sort(number_array)
DEFINE array_size = LENGTH OF number_array
IF array_size <= 1 THEN
RETURN number_array
ENDIF
FOR outer_index = 0 TO array_size - 2
FOR inner_index = 0 TO array_size - outer_index - 2
IF number_array[inner_index] >
number_array[inner_index + 1] THEN
SWAP number_array[inner_index] WITH
number_array[inner_index + 1]
ENDIF
ENDFOR
ENDFOR
RETURN number_array
ENDFUNCTION
Review the following key improvements:
- Descriptive variable names -- number_array vs. arr.
- Proper bounds checking to prevent array overruns.
- Clear nested loop structure with correct termination conditions.
- Explicit SWAP operation instead of cryptic assignments.
- Input validation for array size.
- Consistent indentation demonstrating a logical flow.
The second version of the bubble sort algorithm communicates logic and protects against edge cases. A developer could use this version to follow the sorting process -- from the outer loop controlling passes to the inner loop handling element comparisons.
Keep in mind, however, that pseudocode can't catch implementation-specific issues like memory management or type constraints. In the bubble sort example, the pseudocode shows the sorting logic but doesn't address performance optimizations or space complexity concerns that become critical in real-world applications.
Twain Taylor is a technical writer, musician and runner. Having started his career at Google in its early days, today, Twain is an accomplished tech influencer. He works closely with startups and journals in the cloud-native space.