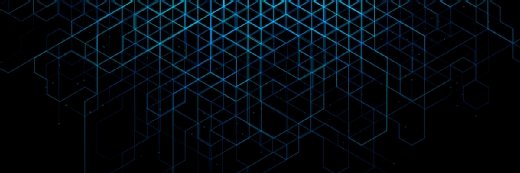
Getty Images
Difference between a statement vs. expression in programming
The decision between statement-centric and expression-oriented languages can influence an individual's programming style and the code's length, complexity and security.
Seemingly minor differences between programming constructs can significantly affect code execution and software development.
Take, for example, statements and expressions. Both make it possible to perform actions within software programs. However, statements typically do not return a value, while expressions do.
Compare sample Rust and Python code excerpts to explore how the distinction in purpose and capabilities between statement-centric languages and more expression-oriented strategies can lead to varying programming approaches.
What is a statement in programming?
A statement is an instruction that performs an action that doesn't compute or generate a value.
Common use cases for statements include the following:
- Declaring a variable.
- Declaring or invoking a function.
- Assigning a value to a variable without requiring computation of the value. For instance, x = 1 because it designates the value of x as a literal -- the integer 1 in this case -- rather than computing a new value and using the variable to store the result.
What is an expression in programming?
An expression is an instruction that computes or evaluates to a new value.
Common uses for expressions include the following:
- Computing a new value. For example, x + 1 is an expression because it computes a new value by incrementing the variable x by one.
- Evaluating the value of an object, such as using the len() function in Python to count the items in an object.
Statement vs. expression: Key differences
Statements and expressions are core building blocks for most programs but work differently. Three key distinctions are as follows:
1. Evaluation outcome
The most important difference between statements and expressions is that statements don't usually generate a direct result or return a value, whereas expressions do.
As an example of what this means in practice, consider the following Python code:
print("Hello")
This is a statement because it executes an instruction. It prints the string "Hello",and doesn't return a value.
In contrast, consider this code:
len("Hello")
This is an expression because it evaluates the number of characters in the string "Hello" and returns a value of 5.
2. Usage
Statements are generally less flexible than expressions. Most programming languages don't allow statements within instructions that need to return a value. Attempting to do this typically generates an error from the compiler.
For example, in the following Python code, the if condition is a statement that controls program flow:
if x > 0:
pass
This is acceptable syntax because the if condition doesn't need to return a value. It only has to evaluate a condition.
Imagine creating a condition that involves computing a new value for x, such as:
if (x + 1) > 0:
pass
The instruction requires including an expression like (x + 1) to complete the evaluation task.
3. Inclusion
It's common to include expressions within statements but not vice versa.
This was the case in the previous example:
if (x + 1) > 0:
pass
The overall instruction is a statement because it doesn't return a value. However, it includes the expression (x + 1) as one step in executing the statement.
Exploring the differences through examples
Let's examine how these differences can manifest in the following Rust and Python code excerpts.
Rust
Rust is an expression-oriented language because most instructions in Rust return values. The following Rust code details a conditional statement that checks whether a number is even or odd based on its divisibility by two:
fn main() {
let number = 10;
// The if expression returns a value, which is assigned to the variable `result`
let result = if number % 2 == 0 {
"even"
} else {
"odd"
};
println!("The number is {}.", result);
}
This function evaluates to either "even" or "odd" and assigns the value to a variable called "result".
Python
Now consider the following Python code:
number = 10
if number % 2 == 0:
print("The number is even.")
else:
print("The number is odd.")
Similar to the Rust example, this Python program uses a conditional if-else statement to evaluate whether the variable "number" is divisible by two. It doesn't assign the resulting output to a new variable. It just prints strings in response to the evaluation. In this respect, the code is not expression-oriented because the overall instruction is a statement, not an expression, even though it includes an expression.
It's possible to turn this code into an expression by assigning the value of the evaluation to a new variable. The process would require extra steps to include additional variable declarations, such as:
result = even
In the Rust example, this additional work isn't necessary. The resulting value is automatically assigned to a variable as an intrinsic part of the evaluation process.
What does this mean for other programming languages?
While some languages are more expression-oriented than others, most languages fall somewhere between the two approaches. For example, Python isn't particularly expression-oriented compared to a language like Rust, where almost everything is an expression rather than a statement. But Python does have some features that make it expression-centric. For example, because Python doesn't require the explicit declaration of variables, it's possible to end up with fewer statements than in a language like Java, where variable declaration is necessary.
Why knowing the difference matters
At first glance, the difference between statements and expressions might not seem important. Yet some programming languages lean more heavily on one type of construct over another and can affect an individual's general programming style and technique. Consider some of the following potential tradeoffs of working with statement-centric vs. expression-oriented languages:
Lines of code
Coding in a language that is more statement-oriented typically inclines developers to adopt an approach that is both imperative and procedural. This means they write out the step-by-step instructions for achieving an intended result, leading, in most cases, to more lines of code. In contrast, code tends to be more compact and declarative with an expression-oriented language.
Security
Arguably, it's easier to avoid oversights that could create security risks within an application that relies primarily on statements rather than expressions. Expressions could assign new values to variables without developers realizing it. In turn, they could create situations where conditions are not correctly evaluated, or policies are not enforced.
This is not to say that expression-oriented languages are inherently less secure. They're not. Developers ultimately bear the responsibility for preventing flaws that could create security issues. But, in certain respects, it is easier to avoid such oversights when using a programming language built around statements rather than expressions.
Complexity
One significant advantage of expression-oriented code is that it tends to be cleaner and more concise than statement-centric language, meaning programs can do more with fewer instructions. However, expression-oriented programming might lead to more complex or buggier code because expressions could result in unintended consequences that cause programs not to execute as developers expect. Like security issues, error-prone or unreliable code suggests more about the user's approach and management than the language itself.
Final takeaway
Instead of focusing on statements vs. expressions in black-and-white terms, understand there's a continuum of approaches for how various languages use each construct.
Chris Tozzi is a freelance writer, research adviser and professor of IT and society who has previously worked as a journalist and Linux systems administrator.