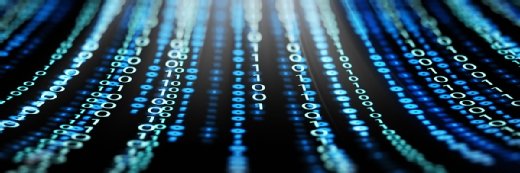
Getty Images
4 pseudocode examples: Python, Java, JavaScript and C++
Successful pseudocode conversion goes beyond simply making the code work. It's about creating implementations that respect each language's conventions.
Diving straight into code can be tempting, but it's also a recipe for head-scratching debugging sessions that can eat up an afternoon.
Taking a step back to outline a strategy in pseudocode offers a chance to spot sneaky edge cases and validate an approach before getting knee-deep in syntax errors. Learn how to transform pseudocode into working implementations across four different programming languages -- Python, Java, JavaScript and C++ -- and review tips for testing and validating the code.
Considerations before pseudocode conversion
When converting pseudocode into source code, developers must navigate the specific rules, syntax, features and quirks that distinguish each programming language. Important areas to watch out for include the following:
- Data types and variables. Depending on the language, a simple variable in the pseudocode might become a string, a char array or even a custom object.
- Standard library integration. Each language comes with a toolkit that dictates how user-friendly it is.
- Error-handling patterns. Languages have distinct ways of handling errors, and a developer's error-handling strategy needs to adapt accordingly.
Adhering to the language's conventions and best practices is essential when translating pseudocode into a target language's source code. The implementation should prioritize readability and maintainability, while considering the language's idioms and performance characteristics. This involves adapting the pseudocode's abstract logic into language-appropriate constructs and data structures.
Starting with pseudocode
Let's tackle a common real-world challenge: validating passwords. A validator must ensure that passwords meet security requirements, while being understandable to users. The following pseudocode example enforces strong password rules.
BEGIN PasswordValidator
// Input validation
INPUT password
SET isValid = FALSE
SET minLength = 8
SET maxLength = 20
SET hasNumber = FALSE
SET hasUpperCase = FALSE
SET hasSpecialChar = FALSE
SET hasConsecutiveChars = FALSE
SET specialChars = "!@#$%^&*"
// Length check (early exit)
IF length(password) < minLength THEN
RETURN "Password too short"
IF length(password) > maxLength THEN
RETURN "Password too long"
// Check consecutive characters (early exit)
FOR i = 0 TO length(password) – 2
IF password[i] equals password[i + 1] THEN
RETURN "Password cannot contain consecutive repeated characters"
// Character checks
FOR EACH character IN password
IF character is digit THEN
SET hasNumber = TRUE
IF character is uppercase THEN
SET hasUpperCase = TRUE
IF character is in specialChars THEN
SET hasSpecialChar = TRUE
// Final validation
IF hasNumber AND hasUpperCase AND hasSpecialChar THEN
RETURN "Password valid"
ELSE
RETURN "Password requirements not met"
END
The validator checks for the minimum and maximum password character length and requires uppercase letters, numbers and special characters, while preventing repeated characters. All of these are common requirements of modern security standards.
Language implementations
The true test of pseudocode lies in its adaptability -- does the core logic translate cleanly across different programming paradigms, while maintaining efficiency and reliability? Different compiler optimizations can affect how these implementations perform.
The following code excerpts take the password validator pseudocode and translate it into working code.
Python implementation
def validate_password(password: str) -> str:
# Initialize validation flags
min_length, max_length = 8, 20
has_number = has_upper = has_special = False
special_chars = "!@#$%^&*"
# Length validation
if len(password) < min_length:
return "Password too short"
if len(password) > max_length:
return "Password too long"
# Check for consecutive characters
for i in range(len(password) - 1):
if password[i] == password[i + 1]:
return "Password cannot contain consecutive repeated characters"
# Character validation
for char in password:
if char.isdigit():
has_number = True
elif char.isupper():
has_upper = True
elif char in special_chars:
has_special = True
# Final validation
if all([has_number, has_upper, has_special]):
return "Password valid"
return "Password requirements not met"
Python's built-in string methods make for an efficient implementation. The isdigit() and isupper() methods eliminate the need for complex character comparisons, while string slicing checks for consecutive characters. Type hints improve code clarity without sacrificing Python's dynamic nature.
Java implementation
public class PasswordValidator {
public static String validatePassword(String password) {
// Constants for validation
final int MIN_LENGTH = 8;
final int MAX_LENGTH = 20;
final String SPECIAL_CHARS = "!@#$%^&*";
// Validation flags
boolean hasNumber = false;
boolean hasUpper = false;
boolean hasSpecial = false;
boolean hasConsecutive = false;
// Length validation
if (password.length() < MIN_LENGTH) {
return "Password too short";
}
if (password.length() > MAX_LENGTH) {
return "Password too long";
}
// Check consecutive characters
for (int i = 0; i < password.length() - 1; i++) {
if (password.charAt(i) == password.charAt(i + 1)) {
return "Password cannot contain consecutive repeated characters";
}
}
// Character validation
for (char c : password.toCharArray()) {
if (Character.isDigit(c)) {
hasNumber = true;
} else if (Character.isUpperCase(c)) {
hasUpper = true;
} else if (SPECIAL_CHARS.indexOf(c) != -1) {
hasSpecial = true;
}
}
// Final validation
if (hasNumber && hasUpper && hasSpecial) {
return "Password valid";
}
return "Password requirements not met";
}
}
Java has a strong type system and built-in character-handling methods, making for straightforward implementations. Java's well-known Character class provides convenient methods, such as isDigit() and isUpperCase() for validation checks. But it's worth noting that the string handling here requires incrementing through each character even as it enables precise control over the validation logic.
JavaScript implementation
function validatePassword(password) {
// Constants
const MIN_LENGTH = 8;
const MAX_LENGTH = 20;
const SPECIAL_CHARS = "!@#$%^&*";
// Length validation
if (password.length < MIN_LENGTH) {
return "Password too short";
}
if (password.length > MAX_LENGTH) {
return "Password too long";
}
// Check consecutive characters using regex
if (/(.)\1/.test(password)) {
return "Password cannot contain consecutive repeated characters";
}
// Character validation using regex
const hasNumber = /\d/.test(password);
const hasUpper = /[A-Z]/.test(password);
const hasSpecial = new RegExp(`[${SPECIAL_CHARS}]`).test(password);
// Final validation
if (hasNumber && hasUpper && hasSpecial) {
return "Password valid";
}
return "Password requirements not met";
}
Developers can use JavaScript's regular expression capabilities to simplify character validation checks. Instead of looping through characters manually, use pattern matching for consecutive character detection and individual character type validation. The code remains readable with cleaner syntax, fewer loops and concise consecutive character detection, while taking advantage of JavaScript's strength in string manipulation.
C++ implementation
string validatePassword(const string& password) {
// Constants
const int MIN_LENGTH = 8;
const int MAX_LENGTH = 20;
const string SPECIAL_CHARS = "!@#$%^&*";
// Validation flags
bool hasNumber = false;
bool hasUpper = false;
bool hasSpecial = false;
// Length validation
if (password.length() < MIN_LENGTH) {
return "Password too short";
}
if (password.length() > MAX_LENGTH) {
return "Password too long";
}
// Increment through password characters
for (size_t i = 0; i < password.length() - 1; i++) {
if (password[i] == password[i + 1]) {
return "Password cannot contain consecutive repeated characters";
}
}
// Character validation
for (const char& c : password) {
if (isdigit(c)) {
hasNumber = true;
} else if (isupper(c)) {
hasUpper = true;
} else if (SPECIAL_CHARS.find(c) != string::npos) {
hasSpecial = true;
}
}
// Final validation
if (hasNumber && hasUpper && hasSpecial) {
return "Password valid";
}
return "Password requirements not met";
}
C++ demands explicit memory handling and type safety. As noted in the sample code excerpt, the const string& parameter prevents unnecessary copying, while standard library functions, such as isdigit() and isupper() from <cctype>, handle character checks efficiently. C++'s standard string methods make the code both safe and performant.
Testing and validation
Pseudocode conversion requires careful verification to ensure logic remains intact across languages. The final executable should maintain consistent validation behavior across platforms. Here's how to approach testing the password validator.
Code review comparison
Review the source code alongside the pseudocode, verifying that each logical block matches its implementation. Pay special attention to edge cases, like passwords with special characters at boundaries or repeated characters in different positions, including boundaries.
Test cases
Create a comprehensive set of test cases that cover the following:
- Passwords that meet all requirements.
- Passwords that fail specific checks, including length, uppercase, numbers and special characters.
- Edge cases, like consecutive characters at different positions in the sequence, whether at the beginning, middle or end of the password string.
- Boundary conditions at minimum and maximum lengths.
Common pitfalls
Watch out for the following language-specific quirks that can affect validation:
- Character encoding differences during each iteration.
- String length calculations that vary across languages.
- Different string comparison behaviors.
- Performance implications of string operations in each language.
Through systematic testing and validation, developers can ensure their pseudocode translations maintain logical integrity across different programming languages. Remember, effective pseudocode conversion creates implementations that respect each language's conventions, while prioritizing security, performance and maintainability.
Twain Taylor is a technical writer, musician and runner. Having started his career at Google in its early days, today, Taylor is an accomplished tech influencer. He works closely with startups and journals in the cloud-native space.